When you visit a website or use an app, have you ever wondered how developers manage the code behind it? Enter Git and GitHub—the ultimate tools for version control and collaboration in software development. Whether you’re a beginner or an experienced developer, understanding Git and GitHub is essential for managing code, collaborating with others, and building a portfolio of projects. In this post, we’ll dive into what Git and GitHub are, how they work, and why they’re indispensable for modern development. By the end, you’ll have a solid understanding of these tools and actionable insights to start using them effectively.
1. What is Git?
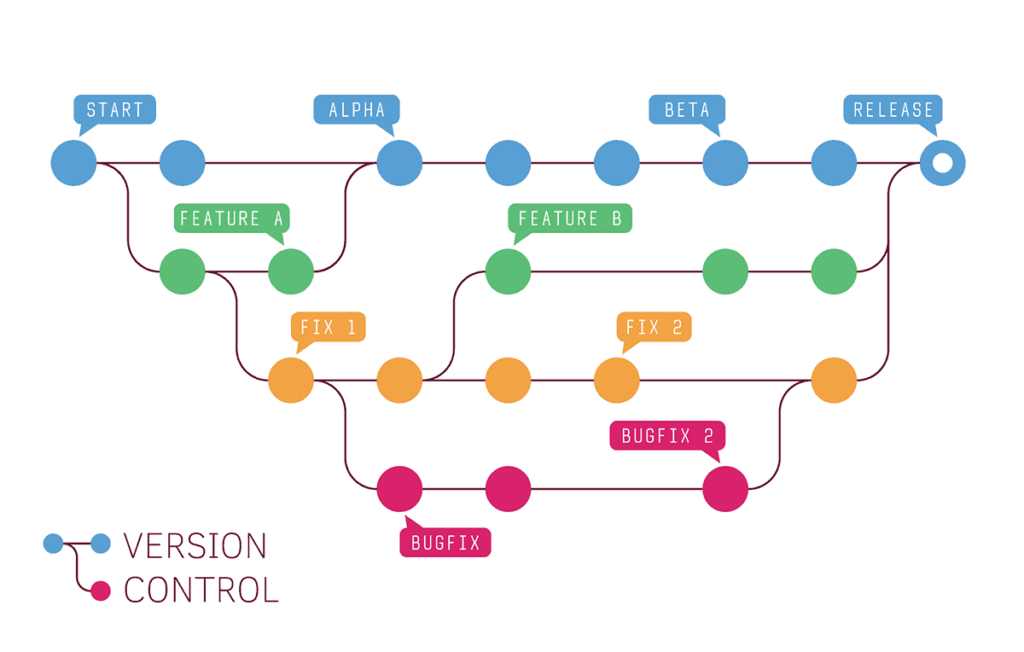
A high-level representation of Git workflow.
Git is a distributed version control system (DVCS) designed to track changes in source code during software development. It allows multiple developers to collaborate on a project efficiently by maintaining a complete history of changes, enabling them to work on different features simultaneously without interfering with each other’s work. Git is known for its speed, flexibility, and robust support for non-linear development workflows, making it the go-to tool for version control in both small and large-scale projects.
Key Concepts:
- Repository (Repo): A folder where your project and its version history are stored. It can be local (on your computer) or remote (on a server like GitHub).
- Commit: A snapshot of your code at a specific point in time. Each commit has a unique ID, a message describing the changes, and a reference to the previous commit.
- Branch: A parallel version of your code, allowing you to work on new features or fixes without affecting the main codebase. The default branch is usually called
main
ormaster
. - Merge: Combining changes from one branch into another. For example, merging a feature branch into the main branch.
- Clone: Creating a local copy of a remote repository.
- Pull: Fetching changes from a remote repository and merging them into your local branch.
- Push: Uploading your local changes to a remote repository.
Example:
Imagine you’re building a website. With Git, you can:
- Save your progress with commits.
- Create a branch to test a new feature.
- Merge the feature into the main codebase once it’s ready.
- Revert to a previous commit if something goes wrong.
2. What is GitHub?
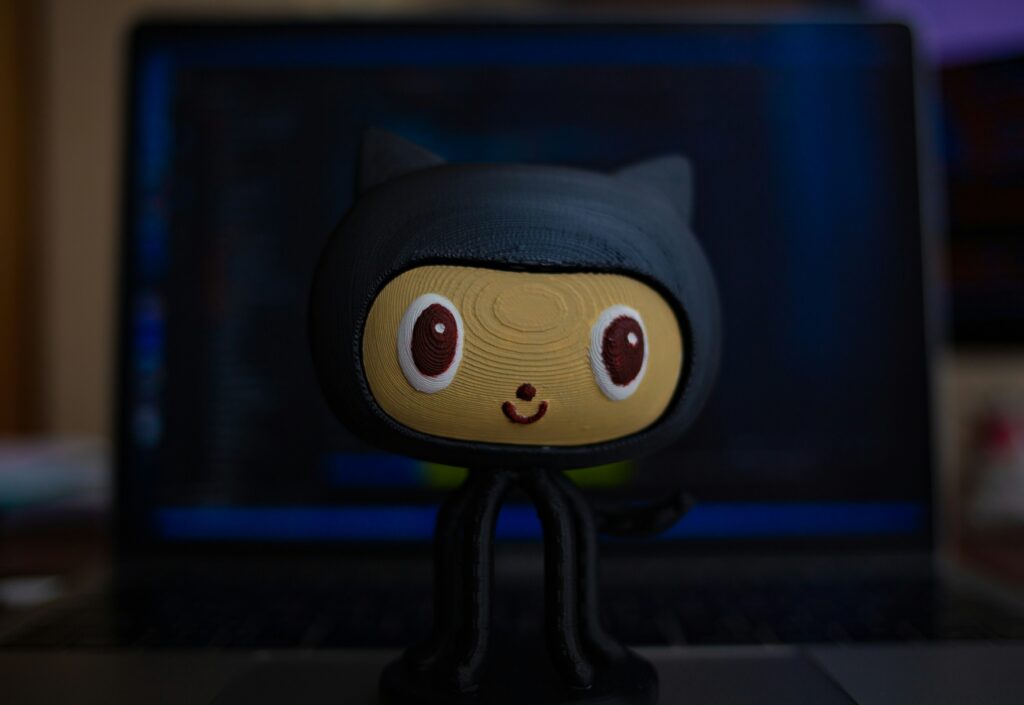
GitHub is a cloud-based platform built on top of Git. It provides a place to host your repositories, collaborate with others, and showcase your work. Think of it as a social network for developers, with features like pull requests, issues, and project management tools.
Key Features:
- Remote Repositories: Store your code in the cloud and access it from anywhere.
- Pull Requests: Propose changes to a project and request that they be merged. This is the backbone of open-source collaboration.
- Issues: Track bugs, feature requests, and tasks. Issues can be assigned, labeled, and linked to pull requests.
- Actions: Automate workflows like testing, building, and deploying your code.
- Projects: Organize tasks and track progress using Kanban-style boards.
- Wiki: Create documentation for your project.
- Pages: Host static websites directly from your repository.
Example:
You’re working on an open-source project. With GitHub, you can:
- Fork the project to create your own copy.
- Make changes and submit a pull request.
- Discuss improvements with the project maintainers.
- Use GitHub Actions to automatically test your code.
3. Git vs. GitHub: What’s the Difference?
While Git and GitHub are often used together, they serve different purposes:
Aspect | Git | GitHub |
---|---|---|
Type | Version control system. | Cloud-based platform for Git. |
Function | Tracks changes to code. | Hosts repositories and enables collaboration. |
Usage | Local operations (e.g., commits). | Remote operations (e.g., pull requests). |
Access | Command-line or GUI tools. | Web-based interface with additional features. |
Ownership | Open-source and free. | Owned by Microsoft; free for public repos, paid for private repos. |
4. Real-World Examples of Git and GitHub in Action
Example 1: Collaborative Development
A team of developers is building a mobile app. They use Git to:
- Create branches for new features.
- Merge changes into the main branch after testing.
- Resolve conflicts when two developers edit the same file.
Example 2: Open-Source Contributions
A developer wants to contribute to an open-source project. They use GitHub to:
- Fork the repository.
- Make changes and submit a pull request.
- Discuss their contribution with the maintainers.
Example 3: Personal Projects
A freelance developer uses Git and GitHub to:
- Track changes to their portfolio website.
- Showcase their work to potential clients.
- Collaborate with other freelancers on shared projects.
5. Common Challenges and How to Overcome Them
Challenge 1: Merge Conflicts
- What Happens: Two developers edit the same file, and Git can’t automatically merge the changes.
- Solution: Use tools like
git diff
to identify conflicts and resolve them manually. Communicate with your team to avoid overlapping work.
Challenge 2: Learning Curve
- What Happens: Beginners may find Git’s command-line interface intimidating.
- Solution: Start with GUI tools like GitHub Desktop or Sourcetree, then gradually learn the commands. Practice with small projects.
Challenge 3: Accidental Changes
- What Happens: You accidentally delete a file or make unwanted changes.
- Solution: Use
git checkout
to revert to a previous commit orgit stash
to save changes temporarily.
Challenge 4: Large Repositories
- What Happens: Cloning and pulling large repositories can be slow.
- Solution: Use shallow clones (
git clone --depth 1
) or tools like Git LFS (Large File Storage) to manage large files.
7. Git Command Cheat Sheet
Here’s a quick reference guide to the most commonly used Git commands. Bookmark this page or print it out for easy access!
🛠️ Basic Commands
# Initialize a new Git repository
git init
# Clone a remote repository
git clone <repository-url>
# Check the status of your repository
git status
# Stage changes for commit
git add <file-name> # Stage a specific file
git add . # Stage all changes
# Commit changes with a message
git commit -m "Your commit message"
# Push changes to a remote repository
git push origin <branch-name>
# Pull changes from a remote repository
git pull origin <branch-name>
🌿 Branching and Merging
# Create a new branch
git branch <branch-name>
# Switch to a branch
git checkout <branch-name>
# Create and switch to a new branch
git checkout -b <branch-name>
# List all branches
git branch
# Merge a branch into the current branch
git merge <branch-name>
# Delete a branch
git branch -d <branch-name>
📜 Viewing History
# View commit history
git log
# View a simplified commit history
git log --oneline
# View changes in a specific commit
git show <commit-hash>
# View changes between two commits
git diff <commit-hash-1> <commit-hash-2>
↩️ Undoing Changes
# Unstage a file
git reset <file-name>
# Revert to the last commit (discard changes)
git checkout -- <file-name>
# Revert to a specific commit
git checkout <commit-hash>
# Amend the last commit
git commit --amend
🌐 Remote Repositories
# Add a remote repository
git it remote add origin <repository-url>
# View remote repositories
git remote -v
# Remove a remote repository
git remote remove origin
# Fetch changes from a remote repository
git fetch origin
8. Best Practices for Using Git and GitHub
a. Write Clear Commit Messages
- Why It Matters: Commit messages are a record of your project’s history. Clear messages make it easier to understand changes and debug issues.
- How to Do It:
- Use the present tense (e.g., “Fix login bug” instead of “Fixed login bug”).
- Be specific and concise. For example:
- Bad: “Update code.”
- Good: “Fix null pointer exception in user authentication.”
- Follow a convention like Conventional Commits:
feat:
for new features.fix:
for bug fixes.docs:
for documentation changes.chore:
for maintenance tasks.
b. Use Branches Strategically
- Why It Matters: Branches allow you to work on new features or fixes without disrupting the main codebase.
- How to Do It:
- Create a new branch for each feature or bug fix.
- Use descriptive branch names like
feature/login
orbugfix/header
. - Follow a branching strategy like Git Flow or GitHub Flow:
- Git Flow: Uses branches like
main
,develop
,feature/
,release/
, andhotfix/
. - GitHub Flow: A simpler model where all changes are made in feature branches and merged into
main
.
- Git Flow: Uses branches like
c. Regularly Pull Changes
- Why It Matters: Pulling changes from the remote repository ensures your local copy is up-to-date and reduces the risk of conflicts.
- How to Do It:
- Use
git pull
regularly to sync your local repository with the remote. - Resolve conflicts immediately if they arise.
- Use
d. Leverage GitHub Features
- Why It Matters: GitHub offers powerful tools for collaboration and project management.
- How to Do It:
- Use issues to track bugs, feature requests, and tasks.
- Enable branch protection rules to prevent accidental changes to the
main
branch. - Use templates for pull requests and issues to standardize communication.
- Take advantage of GitHub Actions to automate workflows like testing and deployment.
e. Keep Your Repository Clean
- Why It Matters: A clean repository is easier to navigate and maintain.
- How to Do It:
- Use a
.gitignore
file to exclude unnecessary files (e.g.,node_modules
,.env
). - Regularly delete merged branches to reduce clutter.
- Use Git LFS (Large File Storage) for large files like images or datasets.
- Use a
f. Review Code Before Merging
- Why It Matters: Code reviews improve code quality and catch bugs early.
- How to Do It:
- Use pull requests to propose changes and request reviews.
- Provide constructive feedback during code reviews.
- Use tools like CodeClimate or SonarQube to automate code quality checks.
g. Document Your Work
- Why It Matters: Documentation helps others understand your code and makes onboarding easier.
- How to Do It:
- Use a README.md file to provide an overview of your project.
- Document your code with comments and docstrings.
- Use GitHub Wiki for more detailed documentation.
h. Test Before You Commit
- Why It Matters: Testing ensures your changes don’t introduce new bugs.
- How to Do It:
- Write unit tests and integration tests for your code.
- Use GitHub Actions or other CI/CD tools to automate testing.
i. Use Tags for Releases
- Why It Matters: Tags mark specific points in your project’s history, such as releases.
- How to Do It:
- Use
git tag
to create a tag for each release. - Follow a versioning scheme like Semantic Versioning (e.g.,
v1.0.0
).
- Use
j. Secure Your Repository
- Why It Matters: Security is critical to protect your code and data.
- How to Do It:
- Use two-factor authentication (2FA) for your GitHub account.
- Avoid committing sensitive information like API keys or passwords. Use environment variables or tools like GitHub Secrets instead.
- Enable code scanning and secret detection to identify vulnerabilities.
Conclusion
Git and GitHub are more than just tools—they’re the foundation of modern software development. Whether you’re working on a solo project or collaborating with a global team, mastering these tools will make you a more efficient and effective developer. So, what are you waiting for? Start exploring Git and GitHub today!
Call-to-Action
- For Beginners: Try creating your first repository on GitHub and making a commit.
- For Experienced Developers: Explore advanced features like GitHub Actions or contribute to an open-source project.
- Discussion Question: What’s your favorite Git or GitHub feature, and how has it helped you in your projects? Share your thoughts in the comments!